Over the last few years I’ve been quietly working on a new operating system called Cosmic OS with several interesting aspects of generative intelligence and artificial consciousness. One of my experiments led to me using PHP and the PHP GD Image Library to create a set of images of random noise. These frames would then be taken by another multiplexer video application like FFmpeg and turn them into something meaningful.
<?php $Width = 1024; $Height = 768; $Image = imagecreatetruecolor($Width, $Height); for($Row = 1; $Row <= $Height; $Row++) { for($Column = 1; $Column <= $Width; $Column++) { $Red = mt_rand(0,255); $Green = mt_rand(0,255); $Blue = mt_rand(0,255); $Colour = imagecolorallocate ($Image, $Red , $Green, $Blue); imagesetpixel($Image,$Column - 1 , $Row - 1, $Colour); } } header('Content-type: image/png'); imagepng($Image); ?>After ensuring it is making the expected images by running
php createrandomnoiseimage.php
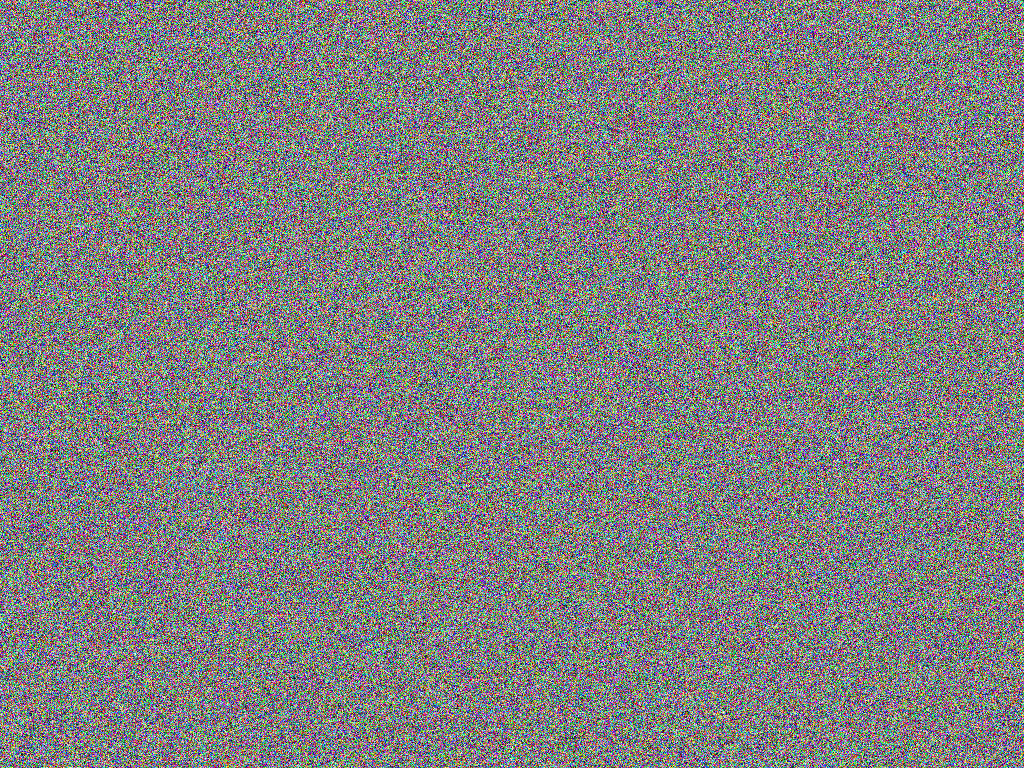
As you can see the PHP GD Library is really awesome, and powerful to produce these kind of images. I’m uising an epic E5-2698 which has 16 cores and can produce one of these images every second. If I was to write a script, I could fork 16 processes at a time by backgrounding them and count the successful_executions() for each running batch command, allowing me to fork the php script to utilise the full cpu resources of the hardware for the application, which is cpu based. With some mucking around in torchvision, the same could be achieved via a gpu ASIC based solution.
#!/bin/bash #generate tha images count=1000 for i in $(seq $count) do php test2.php > images/img"$i".png; done
I am pretty satisfied at the nice set of images this gives me.
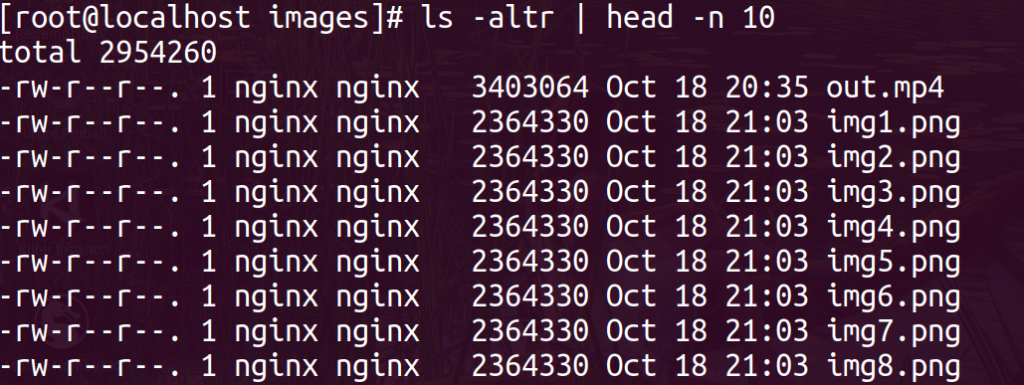
I thought I would be fancy and setup an /images listing path in nginx server. So, if I have my app running at the backend, any of my users could view the progress via the DirectoryIndex listing of the images folder to which the php program outputs the images to. Alternatively I could write another backend application that generated the necessary HTML to provide a ‘status page’ or similar for how the batch jobs are progressing. This is a typical pipeline process you’d expect to see in any professional organisation for the purpose of users checking and monitoring batches of work, and for basic opportunity for secondary applications by utilising modular design and microservice methodologies etc etc.
Notice importantly $uri/ which is necessary for the autoindex on function to work. You can set autoindex off for the location / and you will not need to toggle $uri/ if autoindex isnt used in other paths.
location /images { index index.html index.htm index.php; try_files $uri $uri/ /index.php?$query_string; autoindex on; }
Putting it all together
Now you’ve generated the images and got a way to produce batches and potentially monitor them, we multiplex the video frames into the moving stream.
ffmpeg -framerate 30 -pattern_type glob -i '*.png' -c:v libx264 -pix_fmt yuv420p output.mp4
The mp4 file is indeed a merger of all the screenshots programatically generated by the php-gd library into a moving sequence. This shows how powerful modular software can really be in terms of creating workflow and independent processes. In a real system some kind of database and transactional system could be used which updates the database each time a job was run, and use a mutex to ensure that any job that doesn’t finish is flaggable with a different toggle flag to prevent errors or unexpected job incompletion. Though, that goes a bit beyond the scope of this little tutorial I hope it is useful to someone.
About Cosmic OS and the Future
Cosmic OS utilises a lot of geometric data which is necessary for any serious neural interface or quantum probabilistic interface. I’ve been working hard behind the scenes to achieve this task, although must admit that the technology is a little bit ahead of it’s time. It would be necessary to re-engineer the way that pipelines work in hardware and unix systems to properly improve upon the theories elucidated by Cosmic OS. Many of which utilise convergent quantum probabilistic concepts, and recursive creative geometric and mathematical order functions, some engineering of the basic compiler and hardware would probably be necessary in order to create a computer that produces art and music like a human being.
This investigation into AI, ML and programmatic interfaces, in reference to game theory and quantum electrical systems has been a passion of mine for over a decade, and although the example is simple, do not underestimate the power of such a methodology of working. One day, it will be a commonplace thing for every computer user.
The Impossible Universal Sound Visual Interface
The reason why no universal interface exists, and the square root of minus one isn’t yet used for the development of pipelines and artificially intelligently created hardware and software, is because only such technology has recently become available which makes it mathematically viable to probabilistically represent geometrically the brainwaves of a conscious entity or system without the reliance of real data alone, instead a proper ‘genetic’ programmatic model can be applied which effectively allows all possibilities to be calculated. For example all of the possible 1920×1080 images that can be created, or all of the possible CD or DVD’s that can be created, allows such an approach to produce all music and art ever created, or ever will be created.
Therefore, this study and researches it not to be underestimated, except the models and computer power of man which solely and inadequately fail to properly reach the heights necessary to find 2 to the power of 58 combinations. Ultimately artistic music and drawings is more variable and requires more numerical space than the number of atoms as pixels can be represented in the currently visible universe. A difficult and almost incomprehensible fact, inspires the continuation and the spirit of these writings and musings, and the veritable technology for a new future which is inevitable as its consequence.
This software may provide only a little insight , but to any interested, inspired or enlightened party, it should be more than enough to provide a backdrop to the researches.